New data visualizers can be created by developers by implementing the interface IDataVisualizer. The Data Visualizer is given a set of features that it responds back to the framework if it can visualize the data set. The Data Visualizer is responsible for showing, hiding, and removing itself.
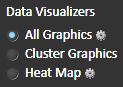
Data Visualizer list
in the framework
IDataVisualizer
The interface IDataVisualizer needs to be implemented and the made exportable through MEF.
Properties
Name | Type | Description |
---|
IsInitialized | bool | Returns to the framework if the data visualizer is initialized. |
Name | string | The name of the data visualizer that is displayed in the framework. |
Methods
Name | Return Type | Description |
---|
CanVisualize(IEnumerable<Feature> data) | bool | Given a set of features the data visualizer must return if it can visualize or not visualize the features. |
Hide() | void | Hides the visualization from the current view. |
Initialize(IDictionary customSettings) | void | Initializes the data visualizer and allows the intake of custom settings from configuration. |
ReloadData(IEnumerable<Feature>data) | void | Reloads the given visualization when the dataset has changed based on a filter or some other action. |
Remove() | void | Removes the data visualizers. If the Data Visualizers is using any GraphicsManagers or other resources that are disposable they should be disposed on this method call. |
Show() | void | Shows the data visualizer. |
Visualize(IEnumerable<Feature>data) | void | Performs the data visualization and shows the results. |
IDialogDataVisualizer
If the Data Visualizer wants to display a dialog to have user controllable settings then it needs to implement the interface IDialogDataVisualizer.
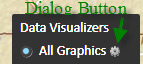
IDialogDataVisualizer Dialog Button
The Data Visualizer has a dialog button next to for DataVisualizers which implement IDialogDataVisualizer.
Methods
Name | Type | Description |
---|
ShowDialog(IEnumerable<Feature> data) | void | The logic that shows the dialog that is associated with the data visualizer should be placed in this method. |
Example
Here's the source code from the FlareClustererVisualizer:
[Export(typeof(IDataVisualizer))]
public class FlareClustererVisualizer : IDataVisualizer
{
private readonly Symbol _symbol;
public FlareClustererVisualizer()
{
_symbol = SymbolManager.GetSymbol("MediumMarkerSymbol");
}
public string Name
{
get { return "Cluster Graphics"; }
}
public bool CanVisualize(IEnumerable<Feature> data)
{
if (data != null && data.Any())
{
if (data.First().Geometry == null)
return false;
return data.First().Geometry.GeometryType.ToUpper() == "ESRIGEOMETRYPOINT" || data.First().Geometry.GeometryType.ToUpper() == "ESRIGEOMETRYPOLYGON";
}
return false;
}
public void Visualize(IEnumerable<Feature> data)
{
LoadFlareClusterer(data);
if (!MapHandler.CurrentMap.Layers.Contains(GraphicsLayer))
{ MapHandler.CurrentMap.Layers.Add(GraphicsLayer); }
}
public void Hide()
{ GraphicsLayer.Visible = false; }
public void Remove()
{
GraphicsLayer.Graphics.Clear();
MapHandler.CurrentMap.Layers.Remove(GraphicsLayer);
}
public void Show()
{ GraphicsLayer.Visible = true; }
public void ReloadData(IEnumerable<Feature> data)
{
GraphicsLayer.Graphics.Clear();
FeatureMap.Clear();
LoadFlareClusterer(data);
}
public bool HasPopup
{
get { return false; }
}
private GraphicsLayer _graphicsLayer;
private GraphicsLayer GraphicsLayer
{
get
{
if (_graphicsLayer == null)
{ _graphicsLayer = new GraphicsLayer { Clusterer = new CustomFlareClusterer { MaximumFlareCount = 9 } }; }
return _graphicsLayer;
}
}
private Dictionary<Graphic, Feature> _featureMap = null;
private Dictionary<Graphic, Feature> FeatureMap
{
get
{
if (_featureMap == null)
{ _featureMap = new Dictionary<Graphic, Feature>(); }
return _featureMap;
}
}
#region private methods
private void LoadFlareClusterer(IEnumerable<Feature> features)
{
var newFeatures = features.Except(FeatureMap.Values);
foreach (var feature in newFeatures)
{
DataContracts.Geometry geometry;
if (feature.Geometry.GeometryType.ToUpper() == "ESRIGEOMETRYPOLYGON")
{ geometry = (DataContracts.Geometry)feature.Geometry.GetCentroid(); }
else
{ geometry = feature.Geometry; }
var g = new Graphic { Symbol = _symbol, Geometry = GeometryTranslator.Translate(geometry) };
//foreach (var kvp in feature.Attributes)
//{ g.Attributes.Add(kvp.Key.Description, kvp.Value); }
FeatureMap.Add(g, feature);
GraphicsLayer.Graphics.Add(g);
}
// uncomment this one to have the map tips show on hover
MapHandler.CurrentMap.MouseMove += new MouseEventHandler(MouseEnter);
// uncomment this one to have the map tips show on click
//MapHandler.CurrentMap.MouseLeftButtonUp += new MouseButtonEventHandler(MouseEnter);
}
private FlareMapTip _currentMapTip = null;
private void MouseEnter(object sender, MouseEventArgs e)
{
if (!(e.OriginalSource is Ellipse) && !(e.OriginalSource is FlareMapTip))
{
if (_currentMapTip != null)
{ _currentMapTip.Hide(); }
return;
}
GeneralTransform generalTransform = MapHandler.CurrentMap.TransformToVisual(Application.Current.RootVisual);
Point transformScreenPnt = generalTransform.Transform(e.GetPosition(MapHandler.CurrentMap));
var graphics = GraphicsLayer.FindGraphicsInHostCoordinates(transformScreenPnt);
var flareMapTip = sender as FlareMapTip;
if (flareMapTip != null)
{ flareMapTip.MapTip_MouseEnter(sender, e); }
var flareClusterer = GraphicsLayer.Clusterer as CustomFlareClusterer;
if (flareClusterer != null)
{
Graphic flare = null;
if (graphics.Count() > 1)
{ flare = flareClusterer.FindGraphicByEllipse(graphics, e.OriginalSource as Ellipse); }
else
{ flare = graphics.FirstOrDefault(); }
if (flare != null)
{
var feature = _featureMap[flare] as Feature;
if (feature != null)
{
feature.IsSelected = true;
MapHandler.MapTip.Show(e);
}
}
}
}
#endregion
/// <summary>
/// Returns true if the data visualizer has been initialized.
/// </summary>
public bool IsInitialized { get; private set; }
/// <summary>
/// Initialize the data visualizer with the provided settings.
/// </summary>
/// <param name="customSettings"></param>
public void Initialize(IDictionary<string, string> customSettings)
{
if (!IsInitialized)
OnInitialize(customSettings);
IsInitialized = true;
}
protected virtual void OnInitialize(IDictionary<string, string> customSettings)
{ }
}
See Also