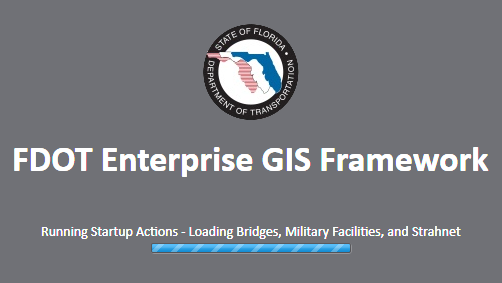
Startup Action Running
Startup Actions are classes that can be configured and are executed on startup of the framework. They will be only run once per a user's session. These actions are executed after all of the map services have been initialized. One example use would be performing a query on bridges and displaying them as a client side graphics before the app starts up for the user. Startup actions are
Configured in Virtual Applications.
IStartupAction
The interface that is implemented for Startup Actions is FDOT.GIS.Client.Domain.IStartupAction.
Properties
Name | Type | Description |
---|
IsInitialized | bool | Indicates if the startup action is initialized |
StartupActionText | string | This is the text that is displayed during the loading screen while the startup action is executing. |
Methods
Name | Return Type | Description |
---|
Initialize(IDictionary customSettings) | void | Indicates if the startup action is initialized. |
ExecuteStartupAction() | void | This is the method where the code that is to be executed for the startup action is placed. |
Events
Name | Description |
---|
Complete | This event is raised to signal the startup action is complete. |
Important: The
event Complete must be raised once the Startup Action is completed. If this event is not raised then the application will be stuck on the loading screen indefinetly.
MEF Exporting
Startup Actions require the MEF attribute [Export(typeof(IStartupAction))] located at the class level to exportable.
Example
[Export(typeof(IStartupAction))]
public class BmsciStartupAction : IStartupAction
{
private GraphicsManager _bridgeGraphicsManager;
private GraphicsManager _militaryGraphicsManager;
private GraphicsManager _roadsGraphicsManager;
private static int _count = 3;
private static object _lockObj = new object();
#region IStartupAction Members
public void Initialize(IDictionary<string, string> customSettings)
{
_militaryGraphicsManager = GraphicManagerFactory.CreateGraphicsManager();
_roadsGraphicsManager = GraphicManagerFactory.CreateGraphicsManager();
_bridgeGraphicsManager = GraphicManagerFactory.CreateGraphicsManager();
}
public void ExecuteStartupAction()
{
MapHandler.DynamicLayers.First().Visible = false;
GetMilitary();
GetRoads();
BridgeManager.BridgesLoaded += BridgeManager_BridgesLoaded;
BridgeManager.LoadBridges();
}
public bool IsInitialized { get; set; }
public string StartupActionText
{
get { return "Loading Bridges, Military Facilities, and Strahnet"; }
}
public event EventHandler Complete;
#endregion
private void CallbackComplete()
{
lock (_lockObj)
{
_count--;
if (_count == 0)
Complete(this, new EventArgs());
}
}
private void BridgeManager_BridgesLoaded(object sender, EventArgs e)
{
List<SolidColorBrush> colors = new List<string>
{
"#FFFF00",
"#FF00FF",
"#808080"
}.Select(c => new SolidColorBrush(ColorConverter.HexColor(c)
)).ToList();
_bridgeGraphicsManager.AddFilteredGeometry(BridgeManager.AllBridges, colors.Select(b => b.Color).ToList(),
"VCLRCD");
CallbackComplete();
}
private void GetMilitary()
{
QueryServiceClient querySvs = ServiceClients.GetQueryServiceClient();
querySvs.QueryCompleted += MilitaryComplete;
var parameters = new SpatialQueryOptions
{
Layer = new Layer {Id = 6},
ReturnAllFields = true,
ReturnGeometry = true,
OutputSpatialReference = MapHandler.MapSpatialReference,
InputSpatialReference = MapHandler.MapSpatialReference,
Criteria = new Criteria
{
ComparisonType = ComparisonType.NotEqual,
Field = new LayerField {Name = "OBJECTID"},
Value = "-1"
}
};
querySvs.QueryAsync(parameters, MapHandler.DynamicLayers.First().Url);
}
private void MilitaryComplete(object sender, QueryCompletedEventArgs e)
{
foreach (Feature f in e.Result.ConvertToFeatures())
{
_militaryGraphicsManager.AddGraphic(f, new SimpleFillSymbol
{
Fill =
new SolidColorBrush(
ColorConverter.
HexColor("#336633"))
}, null);
}
CallbackComplete();
}
private void GetRoads()
{
QueryServiceClient querySvs = ServiceClients.GetQueryServiceClient();
querySvs.QueryCompleted += RoadsComplete;
var parameters = new SpatialQueryOptions
{
Layer = new Layer {Id = 1},
ReturnAllFields = true,
ReturnGeometry = true,
OutputSpatialReference = MapHandler.MapSpatialReference,
InputSpatialReference = MapHandler.MapSpatialReference,
Criteria = new Criteria
{
ComparisonType = ComparisonType.NotEqual,
Field = new LayerField {Name = "OBJECTID"},
Value = "-1"
}
};
querySvs.QueryAsync(parameters, MapHandler.DynamicLayers.First().Url);
}
private void RoadsComplete(object sender, QueryCompletedEventArgs e)
{
List<SolidColorBrush> colors = new List<string>
{
"#FF0000",
"#0000FF",
"#0000FF"
}.Select(c => new SolidColorBrush(ColorConverter.HexColor(c)
)).ToList();
_roadsGraphicsManager.AddFilteredGeometry(e.Result.ConvertToFeatures(), colors.Select(b => b.Color).ToList(),
"COLOR_CODE");
CallbackComplete();
}
}
See Also